Dinero.js
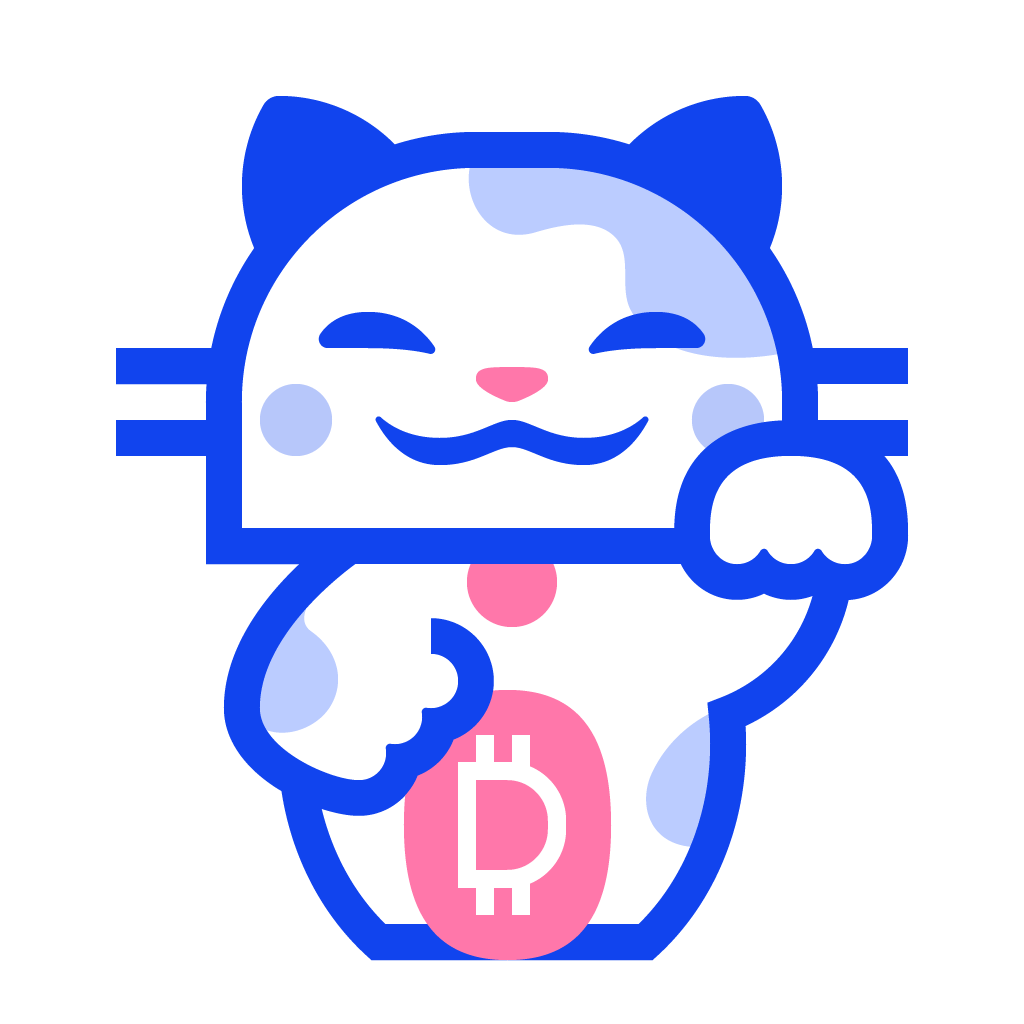
Features
- Immutable and chainable API.
- Global settings support.
- Extended formatting and rounding options.
- Native Intl support (no additional locale files).
- Currency conversion.
Dinero.js v2 is in alpha! Check it out on the
main
branch.
Download/install
Dinero.js provides builds for different environments. It also comes with polyfilled versions for older browsers.
The recommended way of install is via npm or Yarn:
npm install dinero.js --save
// or
yarn add dinero.js
You can also download the files directly or use the jsDelivr CDN.
UMD (browser global)
Include Dinero.js in a script tag and access its methods through the
global Dinero
variable.
<script src="path/to/umd/dinero.js"></script>
<script>
Dinero();
</script>
You can use an alias if you wish:
var Money = Dinero
Any browser that supports the Internationalization API is compatible with Dinero.js. This means most browsers, and Internet Explorer 11 (this one requires the polyfilled version).
CommonJS (Node)
const Dinero = require('dinero.js')
You will need at least Node 6+ with full-icu support.
AMD (RequireJS, System.js, etc.)
requirejs(['path/to/amd/dinero'], function(Dinero) {
//...
})
ES modules (modern browser, Webpack, etc.)
import Dinero from 'path/to/esm/dinero.js'
TypeScript
For Typescript typings, you can use the definition file from DefinitelyTyped.
npm install @types/dinero.js --save
This is a third-party file. Please report issues and open PRs for it on the DefinitelyTyped repository.
React Native
Dinero uses
Number.prototype.toLocaleString
, which by default
isn't bundled with React Native (0.60+) on Android
devices. For formatting and currency symbols to display properly, you need
to change the preferred build flavor of JavaScriptCore in your
project by opening ./android/app/build.gradle
and
changing the line
def jscFlavor = 'org.webkit:android-jsc:+'
to
def jscFlavor = 'org.webkit:android-jsc-intl:+'
.
Quick start
Dinero.js makes it easy to create, calculate and format monetary values in JavaScript. You can perform arithmetic operations, extensively parse and format them, check for a number of things to make your own development process easier and safer.
Note: The library is globally available in the docs for you to be able to test it right in the browser console.
To get started, you need to create a new Dinero instance. Amounts
are specified in minor currency units (e.g.:
"cents" for the dollar). You can also specify an
ISO 4217 currency code
(default is USD
).
This represents β¬50:
const price = Dinero({ amount: 5000, currency: 'EUR' })
You can add or subtract any amount you want, by passing it another Dinero instance:
// returns a Dinero object with amount: 5500
price.add(Dinero({ amount: 500, currency: 'EUR' }))
// returns a Dinero object with amount: 4500
price.subtract(Dinero({ amount: 500, currency: 'EUR' }))
Dinero.js is immutable, which means you'll always get a new Dinero instance when you perform any kind of transformation on it. Your original instance will remain untouched.
price // still returns a Dinero object with amount: 5000
All transformative operations return a Dinero instance, so you can chain methods away as you like:
// returns a Dinero object with amount: 4000
Dinero({ amount: 500 })
.add(Dinero({ amount: 500 }))
.multiply(4)
Note: because method calls are executed
sequentially, mathematical operator precedence doesn't apply. When
you execute the code above, the addition happens before the
multiplication, evaluating to 4000
, while
500 + 500 * 4
would normally evaluate to
2500
. If you need to perform an operation before
another, make sure you call it first.
You can ask all kinds of questions to your Dinero instance. You'll
get a Boolean
in return:
// returns true
Dinero({ amount: 500 }).equalsTo(Dinero({ amount: 500 }))
// returns false
Dinero({ amount: 100 }).isZero()
// returns true
Dinero({ amount: 1150 }).hasCents()
Because Dinero.js uses Number.toLocaleString
under the
hood, you can display it into any format, for any language. But no
need to pass complicated objects of options to format Dinero
instances to your liking. Dinero.js works with intuitive
String
masks:
// returns $5.00
Dinero({ amount: 500 }).toFormat('$0,0.00')
Just set the locale before you call toFormat
, and
you'll get a display result with the proper format:
// returns 5 000 $US
Dinero({ amount: 500000 })
.setLocale('fr-FR')
.toFormat('$0,0')
If you don't want to set the locale all the time, you can also define it globally:
Dinero.globalLocale = 'de-DE'
// returns 5.000 $
Dinero({ amount: 500000 }).toFormat('$0,0')
You can still pass a locale to your Dinero instance if you need, which will prevail over the global one. If you use a transformative method on a Dinero object, its local locale will be inherited.
// returns 10 $US
Dinero({ amount: 500 })
.setLocale('fr-FR')
.add(Dinero({ amount: 500 }))
.toFormat('$0,0')
By default, new Dinero objects represent monetary values with two decimal places. If you want to represent more, or if you're using a currency with a different exponent, you can specify a precision.
// represents $10.545
Dinero({ amount: 10545, precision: 3 })
// The Japanese yen doesn't have sub-units
// this represents Β₯1
Dinero({ amount: 1, currency: 'JPY', precision: 0 })
If you're using the same currency more than once, it might be worth setting a default precision.
// The Iraqi dinar has up to 3 sub-units
Dinero.defaultCurrency = 'IQD'
Dinero.defaultPrecision = 3
// represents IQD1
Dinero({ amount: 1000 })
This is only a preview of what you can do. Dinero.js has extensive documentation with examples for all of its methods.
Contributing
Pull requests are welcome! Please check the contributing guidelines for install instructions and general conventions.
Community
Selected content about Dinero.js:
- JSJ 351: Dinero.js with Sarah Dayan
- Build a Shopping Cart with Vue and Dinero.js
- How to Handle Monetary Values in JavaScript
- Comparison with Numeral.js
- Submit your own blog post/tutorial!
Support
Show some love by upvoting on Product Hunt if you like, support and/or use the library πΌπ
Contributors
This project follows the all-contributors specification.
Acknowledgements
Dinero.js is inspired from Martin Fowler's monetary representation. Design-wise, it draws inspiration from Money PHP, Luxon, Moment.js and Numeral.js (even though it doesn't rely on any of them).
Logo by David DeSandro.
License
Dinero.js is licensed under MIT.